App Drop-in UI Customization
Integrations guide
Layout
Set the corner radius for primary buttons:
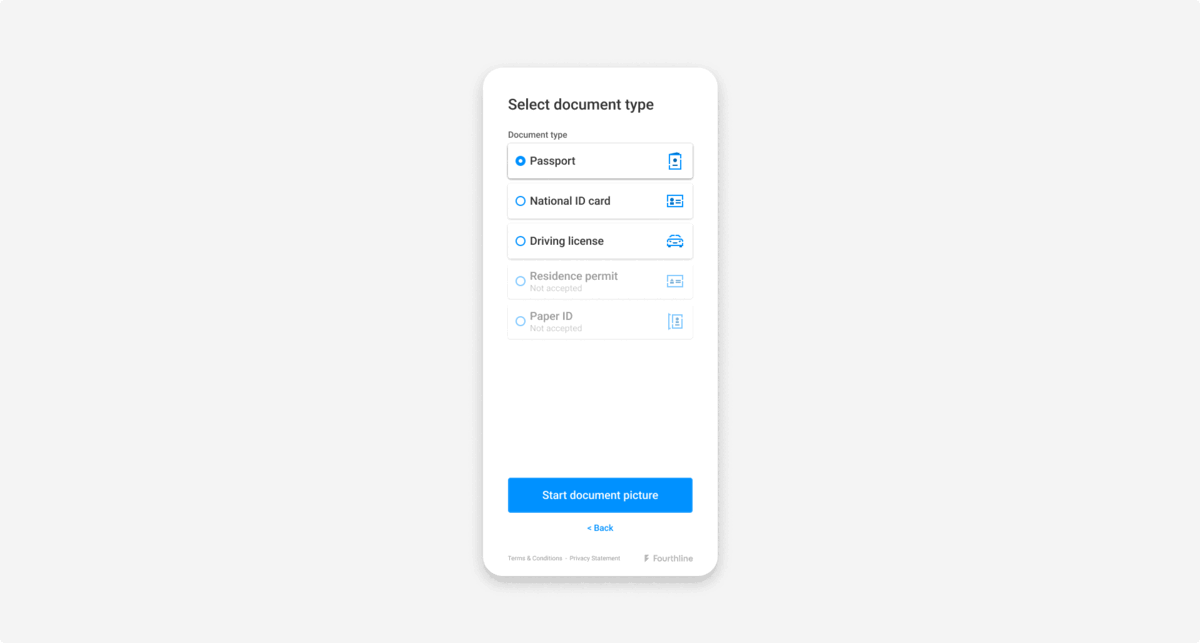
You can set the corner radius as follows:
Property | Description |
---|---|
primaryButtonCornerRadius | The corder radius for primary buttons. |
Android & iOS examples
Example code:
import com.fourthline.orca.core.flavor.OrcaFlavor
import com.fourthline.orca.core.flavor.OrcaLayouts
val customFlavor = OrcaFlavor(
layouts = OrcaLayouts(primaryButtonCornerRadius = 0) // Rectangular buttons
// layouts = OrcaLayouts(primaryButtonCornerRadius = 8) // Buttons with corner radius equal to 8 dp
// layouts = OrcaLayouts(primaryButtonCornerRadius = OrcaLayouts.Round) // Buttons with rounded corners
)
// Usage
import com.fourthline.orca.Orca
import com.fourthline.orca.[product].[Product]CustomizationConfig
import com.fourthline.orca.[product].[product]
val customizationConfig = [Product]CustomizationConfig(flavor = customFlavor)
Orca.[product](context)
...
.customize(config = customizationConfig)
...
var flavor = OrcaFlavor()
flavor.layouts.primaryButtonCornerRadius = .zero // Rectangular buttons
// flavor.layouts.primaryButtonCornerRadius = 8 // Buttons with corner radius equal to 8
// flavor.layouts.primaryButtonCornerRadius = OrcaLayout.CornerRadius.round // Buttons with rounded corners
// Add to the Orca Kyc builder
Orca
.builder
.withDefaultFlow()
.addDataSource(localDataSource)
.addFlavor(flavor)
.present { completionReason in
switch completionReason {
case .completed(let kycInfo):
print("Finished Orca with kyc info: \(kycInfo)")
default: break
}
}
Plugin examples
Example configuration JSON:
{
"primaryButtonCornerRadius": Int
}
primaryButtonCornerRadius
can have the following values:
- Value -1: Button with rounded corners
- Value >= 0: Button with corner radius equal to the value
Example code:
var orcaLayout = `{
"primaryButtonCornerRadius": 8
}`
var config = `{
"flowType": "defaultFlow",
"configuration": {
"flavor": {
"layouts": ${orcaLayout}
}
}
}`
Fourthline.startKyc(
config,
function(kycJson) {
// Extract and process the information from the jsonResult string
var jsonResult = JSON.parse(kycJson);
},
function(error) {
// Extract and process the information from the jsonError string
var jsonError = JSON.parse(error);
}
);
String orcaLayout = '{"primaryButtonCornerRadius": 8}';
String config = """
{
"flowType": "customFlow",
"configuration": {
"flowSteps": ["selfieFlow", "addressFlow"],
"flavor": {
"layouts": $orcaLayout
}
}
}
""";
try {
String result = await _fourthlinePlugin.startKyc(config) ?? "Could not start Orca";
// Extract and process the information from the jsonResult string
print('The success response is: $result');
} on PlatformException catch (e) {
// Extract and process the information from the jsonError string
String error = e.toString();
};
// Instance variable defined in your Dart class
final _fourthlinePlugin = Fourthline();
import { NativeModules } from 'react-native'
var orcaLayout = `{
"primaryButtonCornerRadius": 8
}`
var config = `{
"flowType": "defaultFlow",
"configuration": {
"flavor": {
"layouts": ${orcaLayout}
}
}
}`;
NativeModules.Fourthline.startKyc(config)
.then((kycJson) => {
// Extract and process information from the jsonResult string
var jsonResult = JSON.parse(kycJson);
})
.catch((error) => {
// Extract and process information from the jsonError string
var jsonError = JSON.parse(error.message);
});
Updated 3 months ago
Related pages