App UI Customization
Integrations guide
Fonts
Set the fonts in the UI:
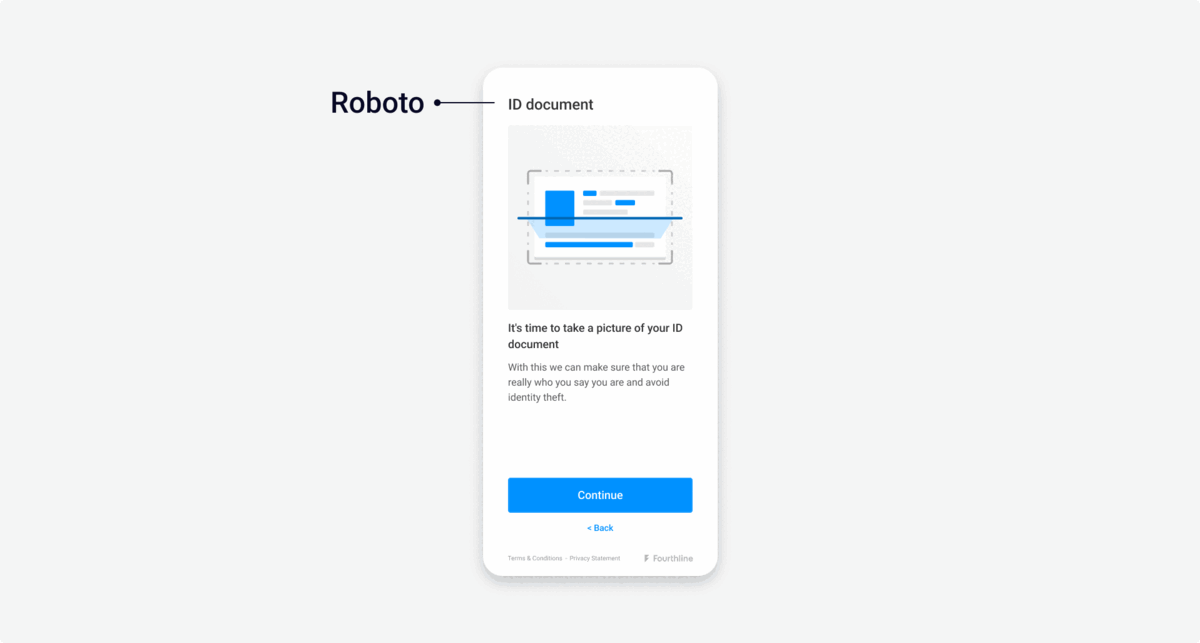
Fonts
You can define fonts as follows:
Property | Description |
---|---|
screenHeader | The main heading on screens. Default: Roboto-Medium 24 |
screenTitle | The subtitle on screens. Default: Roboto-Medium 18 |
screenMessage | The description of the screen. Default: Roboto-Regular 16 |
primaryButton | The font for the primary button. Default: Roboto-Medium 18 |
secondaryButton | The font for the secondary button. Default: Roboto-Medium 14 |
inputField | The font for input fields. Default: Roboto-Medium 18 |
inputFieldPlaceholder | The font for input field placeholders. Default: Roboto-Medium 18 |
inputFieldTitle | The font for input field headings and titles. Default: Roboto-Medium 14 |
inputFieldStatus | The font for input field errors and hints. Default: Roboto-Medium 12 |
scannerInstructionText | The font for scanner instructions. Default: Roboto-Medium 20 |
confirmationScreenTitle | The font for the title of confirmation screens. Default: Roboto-Medium 20 |
confirmationScreenCheckpoints | The font for checkpoints on confirmation screens. Default: Roboto 16 |
tableElementTitle | The font for titles of table view elements. Default: Roboto-Medium 16 |
tableElementDescription | The font for descriptions of table view elements. Default: Roboto 14 |
instructionsLink | The font for linked instructions buttons. Default: Roboto-Medium 14 |
hintText | The font for hint text beneath input fields. Default: Roboto-Regular 14 |
popupHeader | The font for headings on popup screens. Default: Roboto-Medium 24 |
popupMessage | The font for message text on popup screens. Default: Roboto 16 |
popupTitle | The font for error text on popup screens. Default: Roboto-Medium 18 |
Android & iOS examples
Example code:
import com.fourthline.orca.core.flavor.OrcaFlavor
import com.fourthline.orca.core.flavor.OrcaFonts
val customFlavor = OrcaFlavor(
fonts = OrcaFonts(
screenHeader = OrcaFonts.Font.FromFontRes(fontRes = R.font.roboto_medium, size = 20),
primaryButton = OrcaFonts.Font.FromFile(file = File(...), size = 18),
)
)
val customizationConfig = [Product]CustomizationConfig(flavor = customFlavor)
Orca.[product](context)
...
.customize(config = customizationConfig)
...
var flavor = OrcaFlavor()
flavor.fonts.screenHeader = UIFont(name: "Roboto-Medium", size: 22)!
flavor.fonts.primaryButton = UIFont(name: "Roboto-Regular", size: 20)!
// Add to the Orca Kyc builder
let configuration = KycConfig(supportedCountries: localDataSource.supportedCountries)
let customization = KycCustomizationConfig(flavor: flavor)
Orca.kyc
.configure(with: configuration)
.customize(with: customization)
.present { result in
switch result {
case .success(let kycInfo):
print("Finished Orca with kyc info: \(kycInfo)")
case let .failure(kycError):
print("Finished Orca with error: \(kycError)")
}
Plugin examples
Example configuration JSON:
{
"fonts": Orca Fonts Json,
"colors": Orca Colors Json,
"localization": Orca Localization Json,
"layouts": Orca Layouts Json
}
- The font name you set in
OrcaFlavor
must match the one defined in the font file metadata (without the file extension). - Android: Add the otf/ttf font files to the Android project asset folder.
- iOS: Add the custom font files to both the iOS project folder and the application's
Info.plist
file. - All drop-in elements are optional and if not set, the default is used.
{
"orcaElementName": {
"fontName": String,
"fontSize": Int
}
}
Example code:
var orcaFonts = `{
"screenHeader": {
"fontName": "Avenir-Medium",
"fontSize": 24
},
"screenTitle": {
"fontName": "Poppins-Regular",
"fontSize": 18
},
"screenMessage": {
"fontName": "Poppins-Regular",
"fontSize": 16
},
"primaryButton": {
"fontName": "Avenir-Medium",
"fontSize": 16
},
"secondaryButton": {
"fontName": "Poppins-Regular",
"fontSize": 16
},
"instructionsLink": {
"fontName": "Avenir-Light",
"fontSize": 14
},
"hintText": {
"fontName": "Avenir-Light",
"fontSize": 14
},
"inputField": {
"fontName": "Avenir-Light",
"fontSize": 18
},
"inputFieldPlaceholder": {
"fontName": "Avenir-Light",
"fontSize": 18
},
"inputFieldTitle": {
"fontName": "Avenir-Medium",
"fontSize": 14
},
"inputFieldStatus": {
"fontName": "Avenir-Light",
"fontSize": 14
},
"scannerInstructionText": {
"fontName": "Avenir-Medium",
"fontSize": 20
},
"confirmationScreenTitle": {
"fontName": "Avenir-Medium",
"fontSize": 20
},
"confirmationScreenCheckpoints": {
"fontName": "Avenir-Medium",
"fontSize": 16
},
"tableElementTitle": {
"fontName": "Avenir-Light",
"fontSize": 18
},
"tableElementDescription": {
"fontName": "Avenir-Light",
"fontSize": 14
},
"popupHeader": {
"fontName": "Avenir-Medium",
"fontSize": 18
},
"popupTitle": {
"fontName": "Avenir-Light",
"fontSize": 18
},
"popupMessage": {
"fontName": "Avenir-Light",
"fontSize": 16
}
}
`
var config = `{
"flowType": "defaultFlow",
"configuration": {
"flavor": {
"fonts": ${orcaFonts}
}
}
}`;
Fourthline.startKyc(
config,
function(kycJson) {
// Extract and process information from the result String received in JSON format
var jsonResult = JSON.parse(kycJson);
},
function(error) {
// Extract and process information from the error String received in JSON format
var jsonError = JSON.parse(error);
}
);
String orcaFonts = """
{
"screenHeader": {
"fontName": "Avenir-Medium",
"fontSize": 24
},
"screenTitle": {
"fontName": "Poppins-Regular",
"fontSize": 18
},
"screenMessage": {
"fontName": "Poppins-Regular",
"fontSize": 16
},
"primaryButton": {
"fontName": "Avenir-Medium",
"fontSize": 16
},
"secondaryButton": {
"fontName": "Poppins-Regular",
"fontSize": 16
},
"instructionsLink": {
"fontName": "Avenir-Light",
"fontSize": 14
},
"hintText": {
"fontName": "Avenir-Light",
"fontSize": 14
},
"inputField": {
"fontName": "Avenir-Light",
"fontSize": 18
},
"inputFieldPlaceholder": {
"fontName": "Avenir-Light",
"fontSize": 18
},
"inputFieldTitle": {
"fontName": "Avenir-Medium",
"fontSize": 14
},
"inputFieldStatus": {
"fontName": "Avenir-Light",
"fontSize": 14
},
"scannerInstructionText": {
"fontName": "Avenir-Medium",
"fontSize": 20
},
"confirmationScreenTitle": {
"fontName": "Avenir-Medium",
"fontSize": 20
},
"confirmationScreenCheckpoints": {
"fontName": "Avenir-Medium",
"fontSize": 16
},
"tableElementTitle": {
"fontName": "Avenir-Light",
"fontSize": 18
},
"tableElementDescription": {
"fontName": "Avenir-Light",
"fontSize": 14
},
"popupHeader": {
"fontName": "Avenir-Medium",
"fontSize": 18
},
"popupTitle": {
"fontName": "Avenir-Light",
"fontSize": 18
},
"popupMessage": {
"fontName": "Avenir-Light",
"fontSize": 16
}
}
""";
String config = """
{
"flowType": "customFlow",
"configuration": {
"flowSteps": ["selfieFlow", "addressFlow"],
"flavor": {
"fonts": $orcaFonts
}
}
}
""";
try {
String result = await _fourthlinePlugin.startKyc(config) ?? "Could not start Kyc";
// Extract and process information from the result String received in JSON format
print('The success response is: $result');
} on PlatformException catch (e) {
// Extract and process information from the error
String error = e.toString();
};
// Instance variable defined in your Dart class.
final _fourthlinePlugin = Fourthline();
import { NativeModules } from 'react-native'
var orcaFonts = `{
"screenHeader": {
"fontName": "Avenir-Medium",
"fontSize": 24
},
"screenTitle": {
"fontName": "Poppins-Regular",
"fontSize": 18
},
"screenMessage": {
"fontName": "Poppins-Regular",
"fontSize": 16
},
"primaryButton": {
"fontName": "Avenir-Medium",
"fontSize": 16
},
"secondaryButton": {
"fontName": "Poppins-Regular",
"fontSize": 16
},
"instructionsLink": {
"fontName": "Avenir-Light",
"fontSize": 14
},
"hintText": {
"fontName": "Avenir-Light",
"fontSize": 14
},
"inputField": {
"fontName": "Avenir-Light",
"fontSize": 18
},
"inputFieldPlaceholder": {
"fontName": "Avenir-Light",
"fontSize": 18
},
"inputFieldTitle": {
"fontName": "Avenir-Medium",
"fontSize": 14
},
"inputFieldStatus": {
"fontName": "Avenir-Light",
"fontSize": 14
},
"scannerInstructionText": {
"fontName": "Avenir-Medium",
"fontSize": 20
},
"confirmationScreenTitle": {
"fontName": "Avenir-Medium",
"fontSize": 20
},
"confirmationScreenCheckpoints": {
"fontName": "Avenir-Medium",
"fontSize": 16
},
"tableElementTitle": {
"fontName": "Avenir-Light",
"fontSize": 18
},
"tableElementDescription": {
"fontName": "Avenir-Light",
"fontSize": 14
},
"popupHeader": {
"fontName": "Avenir-Medium",
"fontSize": 18
},
"popupTitle": {
"fontName": "Avenir-Light",
"fontSize": 18
},
"popupMessage": {
"fontName": "Avenir-Light",
"fontSize": 16
}
}
`;
var config = `{
"flowType": "defaultFlow",
"configuration": {
"flavor": {
"fonts": ${orcaFonts}
}
}
}`;
NativeModules.Fourthline.startKyc(config)
.then((kycJson) => {
// Extract and process information from the result String received in JSON format
var jsonResult = JSON.parse(kycJson);
})
.catch((error) => {
// Extract and process information from the error String received in JSON format
var jsonError = JSON.parse(error.message);
});
Updated 9 months ago